2.1.5. MsPE for time delay (tau) and dimension (n).
This function implements Multi-scale Permutation Entropy (MsPE) for the selection of n and tau for permutation entropy. Additionally, it only requires a single time series, is robust to additive noise, and has a fast computation time.
- teaspoon.parameter_selection.MsPE.MsPE_n(time_series, delay, m_start=3, m_end=7, plotting=False)[source]
This function returns a suitable embedding dimension, given a time series and embedding delay, based on the dimension normalized MsPE at the optimum delay for a range of dimensions n.
- Parameters:
ts (array) – Time series (1d).
delay (int) – Optimum delay from MsPE.
m_start (int) – minimum dimension in dimension search. Default is 3.
m_end (int) – maximum dimension in dimension search. Default is 8.
- Kwargs:
plotting (bool): Plotting for user interpretation. defaut is False.
- Returns:
n, The embedding dimension for permutation formation.
- Return type:
(int)
- teaspoon.parameter_selection.MsPE.MsPE_tau(time_series, delay_end=200, plotting=False)[source]
This function takes a time series and uses Multi-scale Permutation Entropy (MsPE) to find the optimum delay based on the first maxima in the MsPE plot
- Parameters:
ts (array) – Time series (1d).
delay_end (int) – maximum delay in search. default is 200.
- Kwargs:
plotting (bool): Plotting for user interpretation. defaut is False.
- Returns:
tau, The embedding delay for permutation formation.
- Return type:
(int)
The following is an example implementing the MsPE method for selecting both n and tau:
import numpy as np
from teaspoon.parameter_selection.MsPE import MsPE_n, MsPE_tau
t = np.linspace(0, 100, 1000)
ts = np.sin(t)
m_s, m_e, d_s, d_e = 3, 7, 1, 200
#m_s and m_e are the starting and ending dimensions n to search through
#d_e = max delay tau to search through
#plotting option will show you how delay tau or dimension n were selected
tau = int(MsPE_tau(ts, d_e, plotting = True))
n = MsPE_n(ts, tau, m_s, m_e, plotting = True)
print('Embedding Delay: '+str(tau))
print('Embedding Dimension: '+str(n))
print('Embedding Delay: '+str(tau))
print('Embedding Dimension: '+str(n))
Where the output for this example is:
Embedding Delay: 21
Embedding Dimension: 3
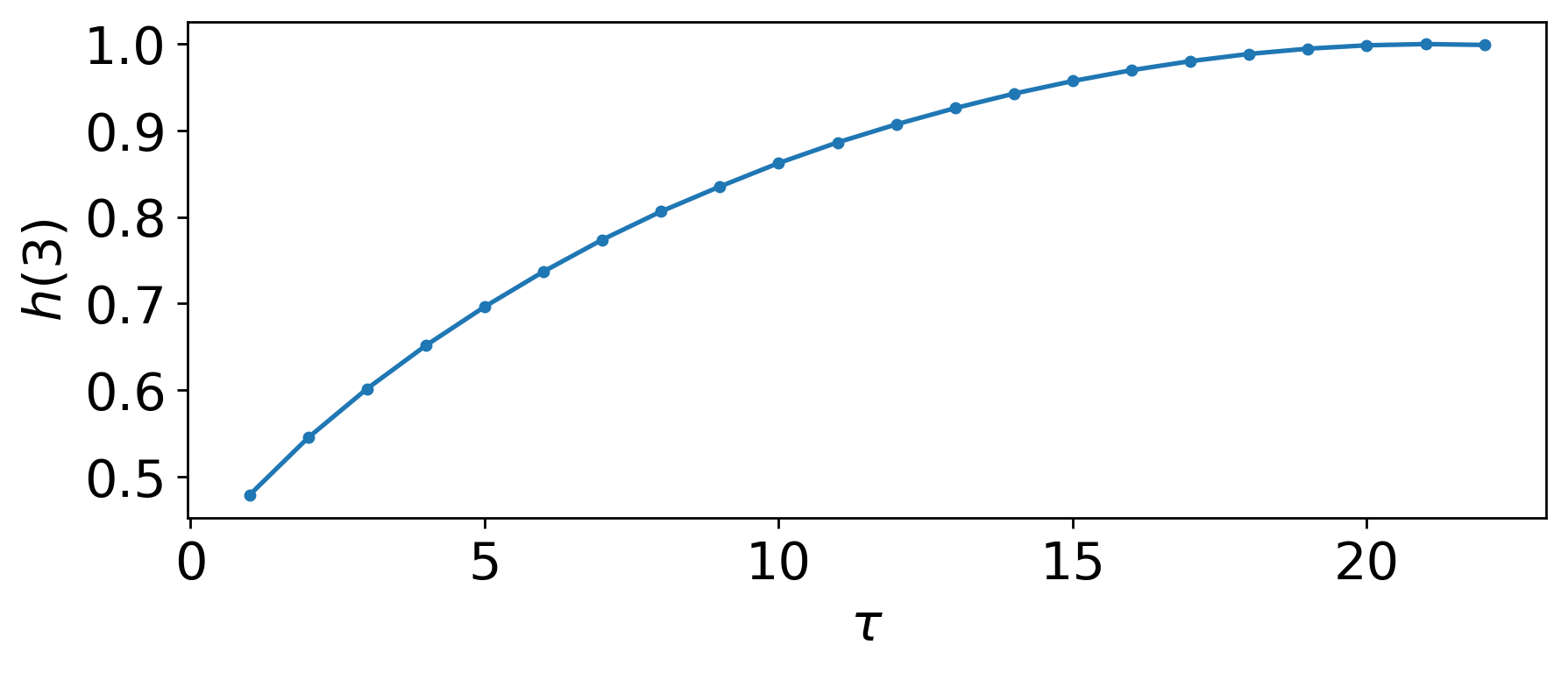
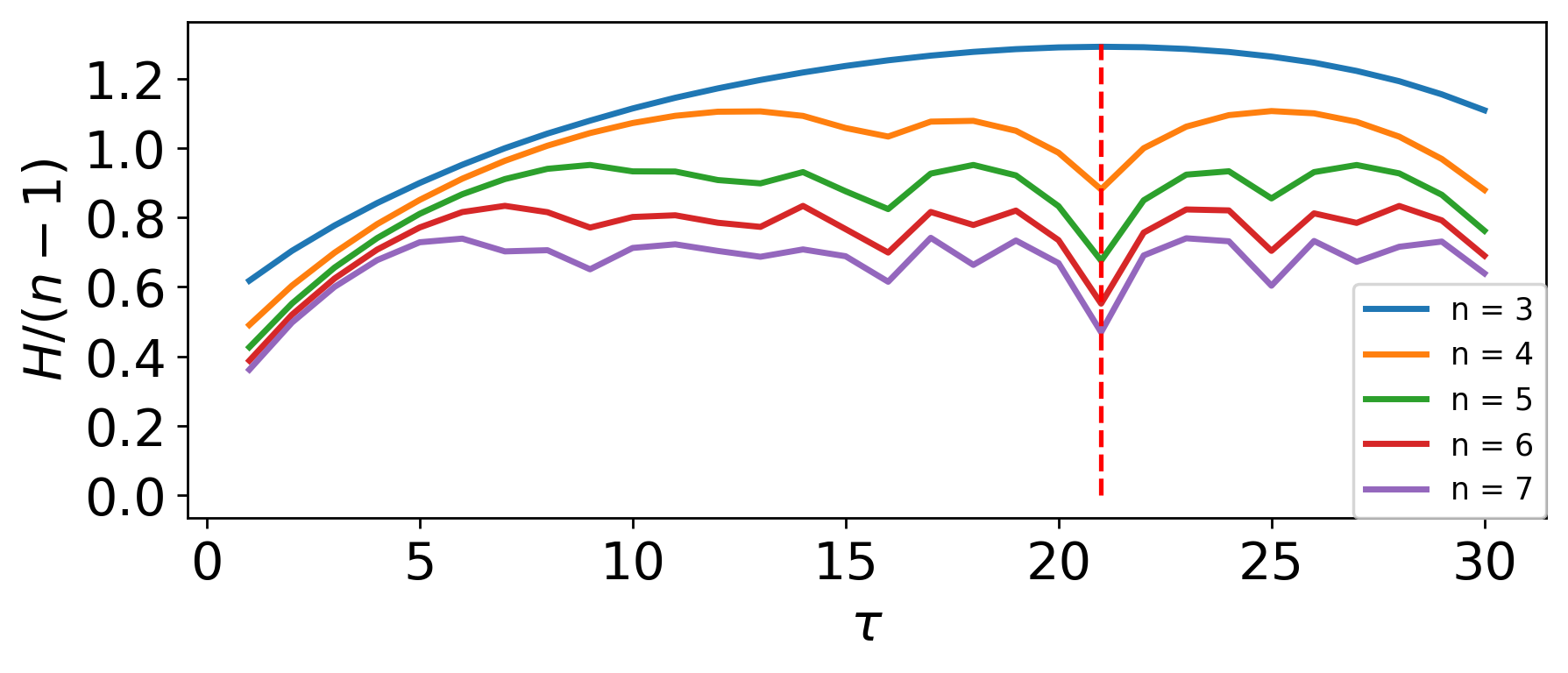